ArrayAccess
接口
平常使用的 Laravel 框架的模型对象既可以使用箭头来进行赋值, 又可以使用数组方式进行赋值.
1 2 3 4 5 6
| <?php
$user = new Users();
$user->name = "RandyChan"; $user['email'] = "M17607475471@163.com";
|
通过以上两种方式都能给对象赋值, 这个功能主要是实现了 ArrayAccess
接口和 __get
和 __set
这两个魔术方法.
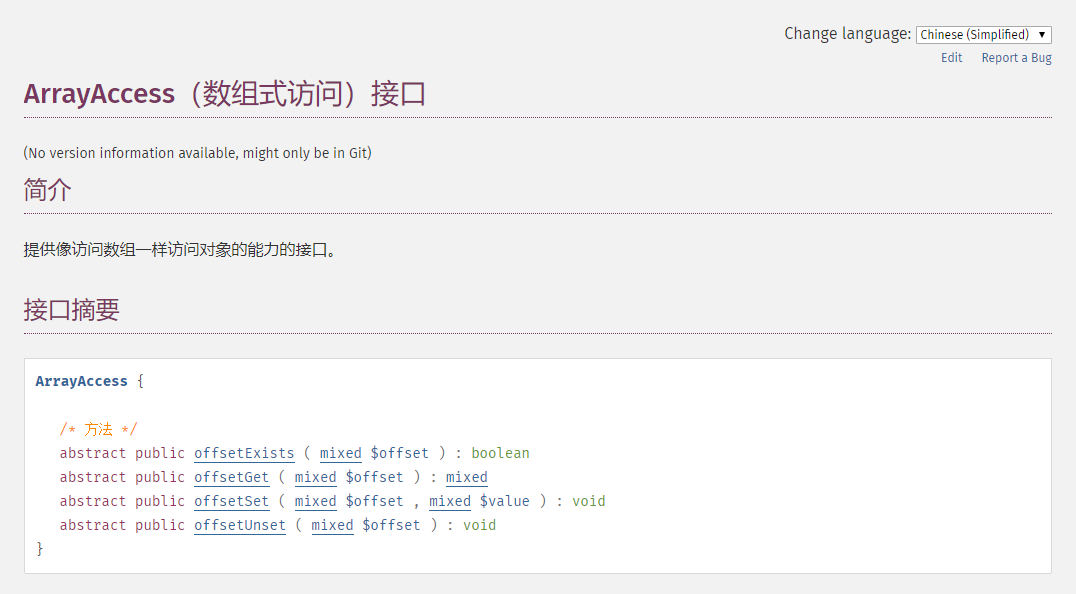
实现 ArrayAccess
接口需要实现四个方法.
offsetExists(mixed $offset): boolean
: 检查一个偏移位置是否存在
offsetGet(mixed $offset): mixed
: 获取一个偏移位置的值
offsetSet(mixed $offset, mixed $value): void
: 设置一个偏移位置的值
offsetUnset(mixed $offset)
: 复位一个偏移位置的值
其中 $offset
参数代表数组的键, $value
参数代表数组的值
实现 ArrayAccess
接口
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77
| <?php
class User implements \ArrayAccess {
private $data = [];
public function offsetExists($offset) { return isset($this->data[$offset]); }
public function offsetGet($offset) { return $this->data[$offset]; }
public function offsetSet($offset, $value) { $this->data[$offset] = $value; }
public function offsetUnset($offset) { if ($this->offsetExists($offset)) { unset($this->data[$offset]); } }
public function __set($offset, $value) { $this->data[$offset] = $value; }
public function __get($offset) { return $this->data[$offset]; } }
$user = new User(); $user['name'] = "RandyChan"; $user->email = "M17607475471@163.com";
echo $user->name . "\n" . $user['email'];
|
执行结果
1 2
| RandyChan M17607475471@163.com
|